[ad#Adobe Banner]
Flex is great for building rich internet applications quickly. Building an application quickly is nice, but to give your application that truly professional touch you need to customize the look and feel of your app. In order to fully customize the look and the feel you will need to use a font that's different than the default serif font that is used everywhere.
The flash player affords you the opportunity to embed many non standard fonts into your app, something that sets it apart from AJAX applications that need to rely on web safe fonts. In the flash IDE this is as easy as setting the font in the properties panel and selecting which character from that font which you want to embed. In a Flex app, things get a little trickier - especially if you want to use a non True Type font, which is the only type it can import natively. However, if you have the Flash IDE you can use its simplicity to get any font you can use in Flash into your Flex app.
The process is broken up into two steps.
- Create a SWF with the font(s) you need embedded in it
- Add Style (CSS) info to your Flex project that imports the font into your Flex project
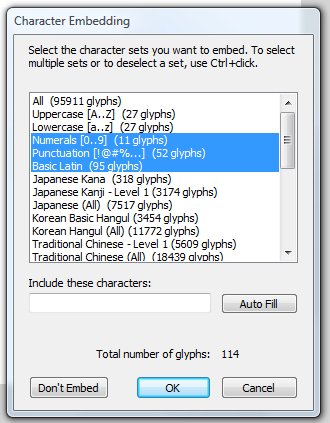
Step one starts with the simplest of FLA files and can be created with earlier versions of the Flash IDE (so you can use Flash 8 or MX2004 if that's all you have). I recommend using a separate FLA for each font you want to embed, that way it is easier to catalog, store and reuse in other projects. Create 4 dynamic text fields. Each text field is for the different versions of the font so you should have:
- normal
- italic
- bold
- bold italic
You need to make sure you set up all 4 text fields to embed all the glyphs from the font you will need. For most western languages Numerals, Punctuation and Basic Latin should cover all the glyphs you will need. Once again, make sure to set up the character embedding for all 4 text fields. If you notice certain parts of your flex document using the default font you may not have set up character embedding. You can of course leave out any of the 4 ( ie italic and italic bold ) that you aren't going to use but if you are going to reuse the SWF I would do all 4.
Once you are done setting the character embedding for all 4 text fields, publish the file to a SWF.
[ad#Google Adsense]
The second step of this process is to add Style to your Flex project that imports the font into the project. That's as simple as adding an @font-face entry to your CSS file or <mx:Style> block. At first you simply give it the path to your font SWF and the name of the font ( ie Futura Book, Calibri ).
@font-face
{
src:url( "path/to/yourFont.swf" );
fontFamily:"Exact Name of Font"
}
You also need to add an @font-face entry for each version of the font you want to use. So if you also wanted to use the bold version you would add the following:
@font-face
{
src: url( "path/to/yourFont.swf");
fontWeight:"bold";
fontFamily:"Exact Name of Font";
}
After you are done adding the appropriate @font-face entries you can style any component you need to or you can style the entire application with your font.
Application
{
fontFamily:"Exact Name of Font";
}
There are a couple other ways to embed fonts, and if you don't have a version of the flash IDE those are the only way to go. If there is enough interest I can cover those in comments or another post. However this is by far the simplest, it allows you the ability to embed non True Type fonts and you can reuse a font more quickly in the future.
[ad#Adobe Banner]
If you liked this post please subscribe to my RSS feed and/or follow me on Twitter. If you only want to see my Flash Friday posts you can follow the subscribe to the Flash Friday feed. How's that for alliteration :) Until next time keep on coding.